Scaling Objects Like a Pro (Without Losing My Sanity)
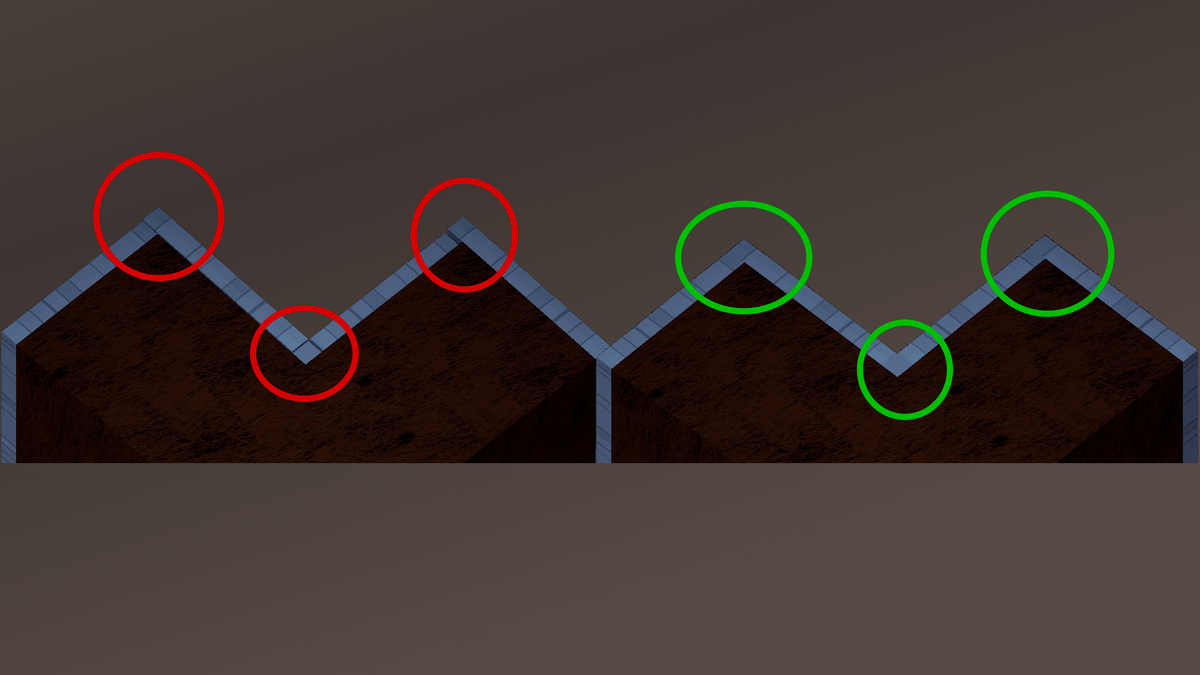
As a solo game developer, I rely heavily on asset packs. While they save me a ton of time (and keep me from embarrassing myself with my 3D modeling skills), they also come with one big issue: scale inconsistencies.
One moment, I’m placing a door, and it looks perfect. The next, I’m placing a table, and suddenly, it looks like it belongs in a dollhouse. Or worse—a chair that would make even a giant feel tiny.
Manually adjusting the scale? Sure, that works. But sometimes, I need objects to be exactly the right size. That’s where the chaos begins:
- Adjusting by eye? Nope, never works.
- Using the Transform scale values? Inconsistent due to random model import settings.
- Screaming at my screen? Temporarily satisfying but ultimately ineffective.
So, I wrote a Scale Adjuster Tool for Unity!
This handy Editor script allows me to select any object in the scene, enter my desired dimensions in meters, and "BAM" perfectly scaled every time. No more guesswork, no more frustration. The script even works seamlessly regardless of the object’s original scale.
Here’s how it works:
- It detects the selected object automatically (no need to drag it into a field).
- It resets the object's scale temporarily to get its real size.
- It calculates the exact scale factor needed to reach the target dimensions.
- It applies the new scale with Unity’s Undo system, so I can revert changes if needed.
No more Alice in Wonderland-sized furniture in my game!
En tant que développeur solo, j’utilise beaucoup de packs d’assets. C’est génial pour gagner du temps (et éviter d’avoir à affronter mes lacunes en modélisation 3D), mais ça vient avec un gros problème : les différences d’échelle.
Un coup, je place une porte, tout est parfait. Puis j’ajoute une table… et là, elle ressemble à un jouet Playmobil. Ou pire, une chaise tellement énorme que même un ogre aurait l’air ridicule dessus.
Ajuster l’échelle à la main ? Mouais… mais parfois, j’ai besoin que les objets soient précisément à la bonne taille. Et c’est là que tout part en vrille :
- À l’œil ? Échec assuré.
- Modifier directement le Transform ? Incohérent à cause des paramètres d’import.
- Hurler devant mon écran ? Satisfaisant sur le moment, mais peu efficace.
Du coup, j’ai écrit un outil d’ajustement d’échelle pour Unity !
Ce script Editor me permet de sélectionner un objet dans la scène, d’entrer ses dimensions cibles en mètres, et hop, il est parfaitement redimensionné. Plus de tâtonnements, plus de frustrations. Il fonctionne même parfaitement, peu importe l’échelle initiale de l’objet.
Voici comment il fonctionne :
- Il détecte l’objet sélectionné automatiquement (pas besoin de le glisser dans un champ).
- Il remet l’échelle à
(1,1,1)
temporairement pour obtenir la vraie taille du modèle. - Il calcule le facteur d’échelle exact pour atteindre la taille cible.
- Il applique la nouvelle échelle en enregistrant l’opération dans Undo (donc je peux revenir en arrière si besoin).
Plus jamais de meubles façon Alice au Pays des Merveilles dans mon jeu !
using UnityEditor;
using UnityEngine;
namespace Editor
{
public class ScaleAdjusterWindow : EditorWindow
{
private Vector3 targetSize = Vector3.one;
[MenuItem("Tools/Scale Adjuster")]
public static void ShowWindow()
{
GetWindow<ScaleAdjusterWindow>("Scale Adjuster");
}
private void OnGUI()
{
GUILayout.Label("Scale Adjustment", EditorStyles.boldLabel);
GameObject selectedObject = Selection.activeGameObject;
if (!selectedObject)
{
EditorGUILayout.HelpBox("Select an object in the scene to adjust its scale.", MessageType.Warning);
return;
}
EditorGUILayout.LabelField("Selected Object: ", selectedObject.name, EditorStyles.boldLabel);
targetSize = EditorGUILayout.Vector3Field("Target Size (meters)", targetSize);
if (GUILayout.Button("Apply Scale"))
{
AdjustScale(selectedObject, targetSize);
}
}
private void AdjustScale(GameObject obj, Vector3 newSize)
{
Renderer renderer = obj.GetComponentInChildren<Renderer>();
if (!renderer)
{
Debug.LogError($"[ScaleAdjuster] No Renderer found on the object {obj.name}!");
return;
}
Vector3 originalScale = obj.transform.localScale;
obj.transform.localScale = Vector3.one;
Vector3 originalSize = renderer.bounds.size;
if (originalSize == Vector3.zero)
{
Debug.LogError($"[ScaleAdjuster] The object {obj.name} has a size of zero!");
obj.transform.localScale = originalScale;
return;
}
Vector3 scaleFactor = new Vector3(
newSize.x / originalSize.x,
newSize.y / originalSize.y,
newSize.z / originalSize.z
);
Undo.RecordObject(obj.transform, "Adjust Scale");
obj.transform.localScale = scaleFactor;
Debug.Log($"[ScaleAdjuster] New scale applied to {obj.name}: {obj.transform.localScale}");
}
}
}